API
Introduction
- The web services available:
- must be called in POST (Content-type x-www-form-urlencoded)
- will return JSON formatted responses
- API access rules :
- please anticipate that ComNpay can add new fields to JSON responses
- some API might need our permission to be used (payment/aliasDebit for example)
HTTPS/SSL
- Web service calls must be encrypted using the TLSv1.1 or TLSv1.2 protocols. The SSLv2, SSLv3 and TLSv1.0 protocols are not authorized.
- The authorized encryption algorithms (ciphers) are the following:
- HIGH: high encryption cipher suites (Algorithms with encryption keys equal to or longer than 128 bits)
- MEDIUM: medium encryption cipher suites
- RSA
- The following encryption algorithms should be prioritised:
- TLS_RSA_WITH_AES_256_CBC_SHA
- TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA
Payment
Debit
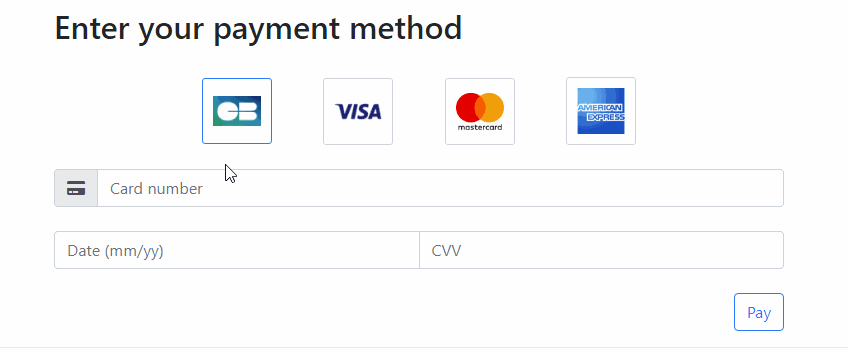
The payment must be set up via tokenization. First of all, a token is generated via the first API call.
This first API call must be made directly on the client side, without going through your server to guarantee cards datas security
You can download an example of this method in Javascript.
DOWNLOAD1-Generating a token
You must generate a token that results from the map information
https://secure.comnpay.com:60000/rest/token/create
POST parameters:
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
cardType | Type of card | CB, VISA, MC |
cardNumber | Card number | Test cards |
cardExpirationDate | Card expiration date | YYMM format |
cvv | Visual cryptogram | |
origin | Origin of payment (URL of your website) | URL to be declared in your URL |
Response
{ "ok": 1, "message": "", "token": { "serialNumber": "VAD-111-111", "tokenRef": "TK1533224578211DEMO364", "cardExpirationDate": "1222", "cardType": "CB", "truncatedCardNumber": "111122XXXXXX4444" } "responseCode": "000" }
2-Payment via token
On the server side, you have to finalize the payment thanks to this previously generated token (do not save the information of the card, only the token is necessary).
https://secure.comnpay.com:60000/rest/payment/tokenDebit
POST parameters:
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
tokenRef | Token previously generated | |
transactionRef | Customer reference number for the transaction | Min length 8 / Max length 40 |
amount | Debit amount | In cents |
force3ds | Forces or prevents use of 3DS | 1 to force 3DS, 0 for the standard case (threshold, geo-filtering) |
redirection3DSV2Url | Mandatory for 3DS V2 | Redirection to achieve the 3D Secure V2 |
browser | Mandatory for 3DS V2 | For mobile applications, the field application is mandatory instead. |
customer | Strongly recommended for 3DS V2 | |
cardholderAccount | Recommended for 3DS V2 | |
caddy | Recommended for 3DS V2 |
3-Finalize debit after 3DS authentication v1
Step 1:
- If 3D Secure interrogation is required (because of threshold or forcing), the payment response will contain:
- The actionCode field with the value AUTH_3DS_REQUISE
- The transaction.verifyEnrollment3dsV1 field to true
- The transaction.verifyEnrollment3dsActionUrl field with the URL to which the cardholder is to be redirected
- The transaction.verifyEnrollment3dsMd and transaction.verifyEnrollment3dsPareq fields in order to push pareq and md information to the authentication page
Example:
{ "ok":1, "message":"ok", "transaction":{ "transactionId":2630, "amount":200, [...] "verifyEnrollment3dsMd":"fUebB6kN11L8qY2v2JeU", "verifyEnrollment3dsPareq":"mS2i8y6R1L60yX6aH3971qN9jX64VwxBbmM9bM4iN9lV7mL5fM4e56pLBeA5xC1wV0dM0lz5pV4jkM4Y6hJ0dOFaW6xE8dU9vDGi", "verifyEnrollment3dsCreq":"", "verifyEnrollment3dsActionUrl":"https://homologation.comnpay.com/3ds.html", "verifyEnrollment3dsV1":true }, "responseCode":"200", "actionCode":"AUTH_3DS_REQUISE" }
Step 2:
- Then redirect your customer to the URL provided by the verifyEnrollment3dsActionUrl parameter, adding the following parameters to your POST request:
- MD
- PaReq
- TermUrl: URL to which the customer will be redirected after 3DS authentication
Exemple :
<form action="https://homologation.comnpay.com/3ds.html" id="3ds" method="post"> <input type="hidden" name="TermUrl" value="http://www.votresite.com/retour3ds.html"/> <input type="hidden" name="MD" value="fUebB6kN11L8qY2v2JeU"/> <input type="hidden" name="PaReq" value="mS2i8y6R1L60yX6aH3971qN9jX64VwxBbmM9b..."/> </form>
Step 3:
During the redirection to your website, you will recover the MD and PaRes parameters in POST. These parameters must then be returned to ComNpay, while finalizing the payment:
https://secure.comnpay.com:60000/rest/payment/end3dsDebit
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
transactionRef | Customer reference of the transaction | Min length 8 |
pares | Value of the “PaRes” field | Returned in the form after 3DS authentication |
md | Value of the “MD” field | Returned in the form after 3DS authentication |
3-Finalize debit after 3DS authentication v2
Step 1:
- If 3D Secure interrogation is required (because of threshold or forcing), the payment response will contain:
- The actionCode field with the value AUTH_3DS_REQUISE
- The transaction.verifyEnrollment3dsV1 field to false
- The transaction.verifyEnrollment3dsActionUrl field with the URL to which the cardholder is to be redirected
- The transaction.verifyEnrollment3dsCreq field in order to push creq information to the authentication page
Example:
{ "ok":1, "message":"ok", "transaction":{ "transactionId":2630, "amount":200, [...] "verifyEnrollment3dsMd":"", "verifyEnrollment3dsPareq":"", "verifyEnrollment3dsCreq":"4DG678VEgfs587DF878sg78sq686T86", "verifyEnrollment3dsActionUrl":"https://homologation.comnpay.com/3ds.html", "verifyEnrollment3dsV1":false }, "responseCode":"200", "actionCode":"AUTH_3DS_REQUISE" }
Step 2:
- Then redirect your customer to the URL provided by the verifyEnrollment3dsActionUrl parameter, adding the following parameter to your POST request:
- creq
The redirection will be done via the URL defined in the redirection3DSV2Url parameter.
Exemple :
<form action="https://homologation.comnpay.com/3ds.html" id="3ds" method="post"> <input type="hidden" name="creq" value="4DG678VEgfs587DF878sg78sq686T86"/> </form>
Step 3:
During the redirection to your website, you will recover the cres parameter in POST. This parameter must then be returned to ComNpay, while finalizing the payment:
https://secure.comnpay.com:60000/rest/payment/end3dsDebit
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
transactionRef | Customer reference of the transaction | Min length 8 |
cres | Value of the “cres” field | Returned in the form after 3DS authentication |
Create alias
Aliases is also created by generating a token fisrt.
https://secure.comnpay.com:60000/rest/alias/tokenCreate
POST parameters:
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
tokenRef | Token generated | |
aliasRef | Customer reference number for the alias | |
origin | Origin of payment (URL of your website) | URL to be declared in your URL |
Debit with alias
Web service for debiting an alias (see card holder).
https://secure.comnpay.com:60000/rest/payment/aliasDebit
POST parameters:
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
transactionRef | Customer reference number for the transaction | Min length 8 / Max length 40 |
alias | Card alias | |
cvv | CVV of the card to ask the customer | You do not have to store it on your application, but ask it to the customer |
amount | Debit amount | In cents |
force3ds | Forces or prevents use of 3DS | 1 to force 3DS, 0 for the standard case (threshold, geo-filtering) |
origin | Origin of payment (URL of your website) | URL to be declared in your URL |
redirection3DSV2Url | Mandatory for 3DS V2 | Redirection to achieve the 3D Secure V2 |
browser | Mandatory for 3DS V2 | For mobile applications, the field application is mandatory instead. |
customer | Strongly recommended for 3DS V2 | |
cardholderAccount | Recommended for 3DS V2 | |
caddy | Recommended for 3DS V2 |
Credits/Refunds
Web service for crediting an initial debit. The amount of the credit must be less than or equal to the initial debit. Several credits can be performed in a single transaction, if the total amount does not exceed the initial debit.
https://secure.comnpay.com:60000/rest/payment/refund
POST parameters:
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
transactionRef | Customer reference number for the transaction | Min length 8 |
amount | Credit amount | In cents |
Payment response
{ "ok": 1, "message": "Paiement accepte", "transaction": { "transactionId": 6145, "amount": 1590, "ok": 1, "confirmed": 1, "cardAlias": "02EF4AFB171F94D2D560A2423BBBE306B0CBB6DE94686918BA0D0E54DB25635F", "cardEndDate": "1612", "cardTruncatedNumber": "111122XXXXXX4444", "cardType": "CB", "binCountryCode": "FR", "transactionDate": "20130628164913", "rejectDate": null, "responseCode": "200", "message": "Paiement accepte", "transactionRef": "1440685092164304", "type": "D", "ask3ds": 0, "auth3dsCode": null, "auth3dsMessage": null, "enrollment3dsCode": null, "enrollment3dsMessage": null, "askAutor": 1, "autorCode": "00", "autorNumber": "1234", "verifyEnrollment3dsMd": null, "verifyEnrollment3dsPareq": null, "verifyEnrollment3dsActionUrl": null, "verifyEnrollment3dsMpi": null, "source": "API", "origin": "mywebsite.com", "initLogin": 10000012345, "memo1Clic": false, "subAccount": null, "customer": { "road": null, "zipCode": null, "email": "tjanvier@comnpay.com", "lastName": null, "country": null, "firstName": null, "quality": null, "phone": null, "city": null, "meetingDate": null, "vatNumber": null, "fiscalCode": null }, "payperlink": { "payperlinkId": "18542911b61b2701873acb9ae25790eb", "paymentType": "D", "beginDate": "20180704161544", "endDate": "20180704161852", "maxQuantity": 1, "language": null, "urlOk": null, "urlNok": null, "productReference": null, "productLabel": null, "amount": 14600, "customer": null, "creationDate": "20180704161544", "deferredPaymentDate": null, "suppressionDate": null, "nbPayments": 1, "template": null, "deadlinePeriod": null, "nextDeadlineDate": null, "deadlineFrequency": 0, "deadlineDay": 0, "timetableEndDate": null } }, "responseCode": "200" }
Transaction search
Transaction search web service
https://secure.comnpay.com:60000/rest/payment/find
POST parameters:
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
transactionRef | Customer reference number for the transaction | Min length 8 / Max length 40 (optional) |
transactionType | Type of transaction | D for debit, C for credit (optional) |
cardAlias | Card alias | (optional) |
startDate | Search start date | Format yyyyMMddHHmmss (optional) |
endDate | Search end date | Format yyyyMMddHHmmss (optional) |
payperlinkId | Id of the payment link | See payperlink creation section |
limit | Limit of the number of transactions | Ignored if <=0 (optional) |
page | Page N°, starting from 1 | Ignored if <=0 (optional) |
Return: list is empty if no results, NULL if access is not authorized. Example:
{ "nbTransactions": 1, "transaction": [ { "transactionId": 6145, "amount": 1590, "ok": 1, "confirmed": 1, "cardAlias": "02EF4AFB171F94D2D560A2423BBBE306B0CBB6DE94686918BA0D0E54DB25635F", "cardEndDate": "1612", "cardTruncatedNumber": "111122XXXXXX4444", "cardType": "CB", "transactionDate": "20130628164913", "message": "Paiement accepte", "responseCode": "200", "transactionRef": "1440685092164304", "type": "D", "binCountryCode": null, "ask3ds": 0, "auth3dsCode": null, "auth3dsMessage": null, "enrollment3dsCode": null, "enrollment3dsMessage": null, "askAutor": 1, "autorCode": "00", "autorNumber": "1234", "verifyEnrollment3dsMd": null, "verifyEnrollment3dsPareq": null, "verifyEnrollment3dsActionUrl": null, "verifyEnrollment3dsMpi": null, "source": "API", "origin": "mywebsite.com", "subAccount": null } ] }
Alias search
Alias search web service
https://secure.comnpay.com:60000/rest/alias/find
Paramètres POST :
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
aliasRef | Customer reference number for the alias | Maximum length 40 (optional) |
transactionType | Type of transaction | D for debit, C for credit (optional) |
cardAlias | Card alias | (optional) |
startDate | Search start date | Format yyyyMMddHHmmss (optional) |
endDate | Search end date | Format yyyyMMddHHmmss (optional) |
limit | Limit of the number of transactions | Ignored if <=0 (optional) |
page | Page N°, starting from 1 | Ignored if <=0 (optional) |
Return: list is empty if no results, NULL if access is not authorized. Example:
{ "nbAliases": 1, "alias": [ { "cardAlias": "02EF4AFB171F94D2D560A2423BBBE306B0CBB6DE94686918BA0D0E54DB25635F", "cardEndDate": "1901", "cardTruncatedNumber": "111122XXXXXX4444", "cardType": "MC", "addDate": "20160905095417", "lastOperationDate": "20160905095433", "aliasRef": "1473062054DEMOSI185", "ok": 1, "confirmed": 1, "message": "Paiement accepte", "responseCode": "200", "askAutor": 1, "autorCode": "00", "autorNumber": "1234" } ] }
Duplicate tickets
- Web services for publishing duplicate tickets in the formats:
- HTML (character string)
- PDF (file encoded in base 64)
https://secure.comnpay.com:60000/rest/receipt/getHtml
https://secure.comnpay.com:60000/rest/receipt/getPdfBase64
POST parameters, identical for the 2 web services:
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
transactionRef | Customer reference number of the transaction | Min length 8 |
Return: character string (HTML text, or file encoded in base 64 for PDF)
Pre-authorization
What is pre-authorization?
This function lets you confirm the validity of a payment card and make sure the customer is solvent, without having debited their account.
- The merchant can take 3 possible actions within the time imparted:
- Actually debit the pre-authorized amount
- Cancel the pre-authorization
- Only use a portion of the amount
Opening a pre-authorization
As with the flow rate, the opening of a pre-authorisation is done via token
https://secure.comnpay.com:60000/rest/preauthorization/tokenOpen
POST parameters:
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
tokenRef | Token previously generated | |
preauthorizationRef | Customer reference number for pre-authorization | Maximum length 40 |
amount | Debit amount | In cents |
force3ds | Forces or prevents use of 3DS | 1 to force 3DS, 0 for the standard case (threshold, geo-filtering) |
redirection3DSV2Url | Mandatory for 3DS V2 | Redirection to achieve the 3D Secure V2 |
browser | Mandatory for 3DS V2 | For mobile applications, the field application is mandatory instead. |
customer | Strongly recommended for 3DS V2 | |
cardholderAccount | Recommended for 3DS V2 | |
caddy | Recommended for 3DS V2 |
Finalize preauthorization after 3DS authentication
Same way as the debit one, with the API:
https://secure.comnpay.com:60000/rest/preauthorization/end3ds
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
preauthorizationRef | Customer reference number for pre-authorization | |
pares | Value of the “PaRes” field | Returned in the form after 3DS authentication |
md | Value of the “MD” field | Returned in the form after 3DS authentication |
Opening a pre-authorization from a card alias
https://secure.comnpay.com:60000/rest/preauthorization/aliasOpen
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
preauthorizationRef | Customer reference number for pre-authorization | Maximum length 40 |
alias | Card alias | |
cvv | Visual cryptogram | Mandatory for 3DS authentication |
amount | Pre-authorization amount | In cents |
origin | Origin of payment (URL of your website) | URL to be declared in your URL |
redirection3DSV2Url | Mandatory for 3DS V2 | Redirection to achieve the 3D Secure V2 |
browser | Mandatory for 3DS V2 | For mobile applications, the field application is mandatory instead. |
customer | Strongly recommended for 3DS V2 | |
cardholderAccount | Recommended for 3DS V2 | |
caddy | Recommended for 3DS V2 |
Closing a pre-authorization
https://secure.comnpay.com:60000/rest/preauthorization/close
POST parameters:
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
fileNumber | Pre-authorization case N° created | Returned in the response of the opening web service |
preauthorizationRef | Customer reference number for pre-authorization | Maximum length 40 |
amount | Debit amount | In cents. If 0, case is cancelled |
Pre-authorization response
{ "ok": 1, "message": "Paiement accepte", "preauthorization": { "preauthorizationId": 247, "fileNumber": "PA20150827000247", "amount": 1590, "status": "CLOSURE_OK", "cardAlias": "02EF4AFB171F94D2D560A2423BBBE306B0CBB6DE94686918BA0D0E54DB25635F", "cardEndDate": "1612", "cardTruncatedNumber": "111122XXXXXX4444", "cardType": "CB", "openDate": "20150827162645", "closureDate": "20150827162726", "autorCode": "00", "autorNumber": null, "message": "Paiement accepte", "responseCode": "200", "preauthorizationRef": "1440685605313683", "transactions": [ { "transactionId": 6146, "amount": 1590, "ok": 1, "confirmed": 1, "cardAlias": "02EF4AFB171F9...D0E54DB25635F", "cardEndDate": "1612", "cardTruncatedNumber": "111122XXXXXX4444", "cardType": "CB", "transactionDate": "20150827162726", "message": "Paiement accepte", "responseCode": "200", "transactionRef": "1440685646812DEMOSI344", "type": "PA", "binCountryCode": null, "ask3ds": 0, "auth3dsCode": null, "auth3dsMessage": null, "enrollment3dsCode": null, "enrollment3dsMessage": null, "askAutor": 1, "autorCode": "00", "autorNumber": "1234", "verifyEnrollment3dsMd": null, "verifyEnrollment3dsPareq": null, "verifyEnrollment3dsActionUrl": null, "verifyEnrollment3dsMpi": null, "source": "API", "origin": "mywebsite.com", "subAccount": null } ], "subAccount": null }, "responseCode": "200" }
Pre-authorization status
- List of possible pre-authorization statuses:
- OPEN_OK: opening of pre-authorization accepted
- OPEN_KO: opening of pre-authorization refused
- CLOSURE_OK: closure of pre-authorization accepted
- CLOSURE_KO: closure of pre-authorization refused (new attempt at closure permitted)
- ABORT: pre-authorization aborted (by the merchant)
Pre-authorization search
Pre-authorization search web service
https://secure.comnpay.com:60000/rest/preauthorization/find
POST parameters:
Parameter | Description | Comments |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
fileNumber | Pre-authorization case N° | E.g.: PA20130701000015 (optional) |
preauthorizationRef | Pre-authorization customer reference number | (optional) |
startDate | Search start date | Format yyyyMMddHHmmss (optional) |
endDate | Search end date | Format yyyyMMddHHmmss (optional) |
limit | Limit for the number of pre-authorizations | Ignored if <=0 (optional) |
page | Page N°, starting from 1 | Ignored if <=0 (optional) |
Return: list is empty if no results, NULL if access is not authorized. Example:
{ "nbPreauthorizations": 1, "preauthorization": [ { "preauthorizationId": 247, "fileNumber": "PA20150827000247", "amount": 1590, "status": "CLOSURE_OK", [...] "transactions": [ { "transactionId": 6146, "amount": 1590, "ok": 1, [...] } ], "subAccount": null } ] }
Payperlink
Payperlink creation
Payperlink creation web service
Find the same functionality on your Afone Paiement backoffice (Paypermail > Generate a payment link)
https://secure.comnpay.com:60000/rest/payperlink/create
POST parameters:
Parameter | Description | Commentary |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
paymentType | Payment type | D, PA, P3F, ALS, SUBD, SUBS, SDD, SDDR |
productReference | Product reference | |
productLabel | Product label | |
transactionRef | Transaction reference | Min length 8 / Max length 40 (35 for SDD/SDDR) |
amount | Amount (optional) | If no amount, the customer will fill the amount to pay |
maxQuantity | Maximum numbers of accepted payments | |
beginDate | Begin date of the payperlink | Example: 20180701000000 |
endDate | End date of the payperlink | Example: 20180731000000 |
language | Payment page language | fr/en/it |
urlOk | Redirection URL after an accepted payment | |
urlNok | Redirection URL after a declined payment | |
template | Payment page template | See Afone Paiement > configuration > customization |
customer | Strongly recommended for 3DS V2 | |
customerReference | Client reference | |
siteReference | Site reference | |
origin | Origin of payment (URL of your website) | URL to be declared in your URL |
transferDate | SEPA transfer date for SDD/SCT | Example: 20180806000000 |
sepaLabel | SEPA operation label (Ustrd) | 140 characters maximum |
urlIpn | IPN URL for transaction confirmation | |
iban | Client IBAN if known (for pre-filling) | For payment types SDD, SDDR / maxQuantity should be set at 1 |
Response
{ "ok": 1, "message": "", "actionUrl": null, "payperlink": { "paymentType": "D", "beginDate": null, "endDate": "20180701000000", "maxQuantity": 1, "language": "en", "urlOk": null, "urlNok": null, "productReference": "TESTREF00001", "amount": 1990, "customer": null, "link": "https://campagne.comnpay.com/f.html?t=3e72785470347c551ad95e457dl5qbf8", "payperlinkId": "3e72785470347c551ad95e457dl5qbf8", "deadlinePeriod": null, "nextDeadlineDate": null, "deadlineFrequency": 0, "deadlineDay": 0, "timetableEndDate": null, "creationDate": "20180605155948", "deferredPaymentDate": null, "suppressionDate": null, "nbPayments": 0, "template": null, "modelForm": null, "productLabel": "Product description" }, "responseCode": "000" }
Payperlink closure
Payperlink closure web service. Link will no longer be available.
https://secure.comnpay.com:60000/rest/payperlink/close
POST parameters:
Parameter | Description | Commentary |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
payperlinkId | Payperlink id generated | Ex : 3e72785470347c551ad95e457dl5qbf8 |
Response
{ "ok": 1, "message": "", "actionUrl": null, "payperlink": { "paymentType": "D", "beginDate": null, "endDate": "20180606113628", "maxQuantity": 1, "language": "en", "urlOk": null, "urlNok": null, "productReference": "TESTREF00003", "amount": 1990, "customer": null, "link": "https://campagne.comnpay.com/f.html?t=3e72785470347c551ad95e457dl5qbf8", "payperlinkId": "3e72785470347c551ad95e457dl5qbf8", "deadlinePeriod": null, "nextDeadlineDate": null, "deadlineFrequency": 0, "deadlineDay": 0, "timetableEndDate": null, "creationDate": "20180605155948", "deferredPaymentDate": null, "suppressionDate": null, "nbPayments": 0, "template": null, "modelForm": null, "productLabel": "Product description" }, "responseCode": "000" }
Payperlink research
Payperlinks research web service (limit of 1000 results)
https://secure.comnpay.com:60000/rest/payperlink/find
POST parameters:
Parameter | Description | Commentary |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
payperlinkId | Payperlink id (optional) | Ex: 3e72785470347c551ad95e457dl5qbf8 |
Response
{ "payperlinks": [ { "paymentType": "D", "beginDate": null, "endDate": "20180701000000", "maxQuantity": 1, "language": "en", "urlOk": null, "urlNok": null, "productReference": "TESTREF00001", "amount": 1990, "customer": null, "link": "https://campagne.comnpay.com/f.html?t=3e72785470347c551ad95e457dl5qbf8", "payperlinkId": "3e72785470347c551ad95e457dl5qbf8", "deadlinePeriod": null, "nextDeadlineDate": null, "deadlineFrequency": 0, "deadlineDay": 0, "timetableEndDate": null, "creationDate": "20180605155948", "deferredPaymentDate": null, "suppressionDate": null, "nbPayments": 0, "template": null, "modelForm": null, "productLabel": "Product description" } ], "nbPayperlinks": 1 }
Payment in 3 installments
P3F research
Payment in 3 times research web service
https://secure.comnpay.com:60000/rest/pnfFile/find
POST parameters:
Parameter | Description | Commentary |
---|---|---|
serialNumber | Serial N° of the virtual terminal | |
key | Security key | |
fileNumber | P3F file number | Ex PNF2016041415997 |
transactionRef | 1st transaction reference | Min length 8 |
amount | File total amount | |
cardNumber | Truncated card number | |
status | Folder status | REFUSED/PENDING/EXPERTISE/ERROR/CLOSED/CANCELED |
startDate | Search start date | Format yyyyMMddHHmmss (optional) |
endDate | Search end date | Format yyyyMMddHHmmss (optional) |
limit | Limit of the number of transactions | Ignored if <=0 (optional) |
page | Page N°, starting from 1 | Ignored if <=0 (optional) |
Réponse
{ "nbPnfFiles": 1, "pnfFile": [ { "fileNumber": "PNF2018071415445", "amount": 17980, "cardAlias": "02EF4AFB171F...B25635F", "cardEndDate": "2006", "cardTruncatedNumber": "111122XXXXXX4444", "entryDate": "20180714163358", "statusDate": "20180813164601", "status": "PENDING", "initLogin": null, "pnfTransactions": [ { "term": 1, "amount": 5993, "ok": 1, "canceled": 0, "cardAlias": "02EF4AFB171F...B25635F", "cardEndDate": "2006", "cardTruncatedNumber": "111122XXXXXX4444", "cardType": "CB", "plannedDate": "20180714163358", "transactionDate": "20180714163517", "message": "Paiement accepte", "responseCode": "200", "retries": 1 }, { "term": 2, "amount": 5993, [...] }, { "term": 3, [...] } ], "customer": { "email": "tjanvier@comnpay.com", [...] } } ] }
Return codes
Code | Description |
---|---|
000 | Processing ok |
001 | Service unavailable |
002 | Invalid configuration |
003 | Data volume exceeded |
004 | Too many attempts |
005 | Required field missing |
006 | Unreadable file |
007 | Request out of time |
008 | Duplicate request |
009 | Invalid parameter |
010 | Access denied |
011 | IP denied |
012 | Account closed |
013 | Action prohibited |
040 | Unknown domain |
060 | Unknown campaign |
061 | Error during e-mail sending |
062 | Error during sms sending |
070 | Invalid cardholder data |
071 | Basket data invalid |
110 | Security ID unknown |
111 | Error: activation VAD |
200 | Payment accepted |
201 | Service unavailable |
202 | Double transactionRef |
203 | Type of transaction invalid |
204 | Currency invalid |
205 | Amount invalid |
206 | Card alias unknown |
207 | Card refused |
208 | Card prohibited |
2081 | Prepaid cards not allowed |
209 | Card invalid |
210 | Card expired |
211 | The expiry date of the card is invalid |
212 | The holder is not 3DS compatible |
213 | Card type invalid |
214 | Transaction unknown |
215 | Card on grey list |
216 | Card on black list |
217 | Maximum amount for virtual EPT reached |
218 | Guarantee refused |
2181 | Guarantee refused after examination |
219 | Guarantee examination in progress |
220 | No transaction in progress for the holder |
221 | Holder authentication refused |
222 | Holder not registered with 3DS |
223 | 3DS in progress |
224 | 3DS incident, information incomplete (md and/or pares missing) |
225 | An incident occurred during 3DS authentication |
226 | 3DSv1 prohibited |
230 | Auto return: technical incident |
231 | Auto return: customer prohibited |
232 | Auto return: bank refusal |
233 | CVV missing |
240 | Pre-authorization accepted |
241 | Case unknown |
242 | Closure prohibited |
243 | Case closed |
244 | Case cancelled |
245 | Closure amount incorrect |
246 | Double pre-authorization reference |
250 | PNF prohibited |
251 | Maximum number of outstanding PNFs reached |
252 | Maximum number of outstanding holder PNFs reached |
253 | PNF case unknown |
254 | Advance of funds already paid |
255 | Outstanding PNF amount reached |
256 | Outstanding holder PNF amount reached |
257 | PNF amount too high |
260 | No initial debit success |
261 | Credit amount invalid |
262 | Maximum monthly credit reached |
263 | Daily balance insufficient |
264 | Credit period exceeded |
270 | Double token reference |
271 | Token unknown |
272 | Alias created |
280 | Session expired |
281 | Transaction canceled |
282 | Abandoned transaction |
299 | Transaction in progress |
301 | Maximum daily number of holder outstandings reached |
302 | Maximum daily amount of holder outstandings reached |
303 | IP BIN country inconsistent |
304 | Country BIN blocked |
305 | Country IP blocked |
306 | Excessive amount |
307 | Maximum daily number of outstandings reached |
308 | Maximum daily amount of outstandings reached |
309 | Maximum monthly number of holder outstandings reached |
310 | Maximum monthly amount of holder outstandings reached |
311 | Maximum monthly number of outstandings reached |
312 | Maximum monthly amount of outstandings reached |
313 | Period between holder transactions too short |
314 | Maximum number of outstandings per minute reached |
315 | Non-French card prohibited with ETerminal |
316 | Number of cards per month reached |
320 | Cardholder declined |
321 | Bin blocked |
322 | Daily maximum number transactions reached by IP |
350 | Domestic transfer amount reached |
351 | International transfer amount reached |
352 | Daily maximum amount of domestic transfers reached |
353 | Daily maximum amount of international transfers reached |
354 | Daily maximum number of domestic transfers reached |
355 | Daily maximum number of international transfers reached |
356 | Monthly maximum amount of domestic transfers reached |
357 | Monthly maximum amount of international transfers reached |
358 | Monthly maximum number of domestic transfers reached |
359 | Monthly maximum number of international transfers reached |
360 | Daily maximum amount of domestic transfers by IBAN reached |
361 | Daily maximum amount of international transfers by IBAN reached |
362 | Daily maximum number of domestic transfers by IBAN reached |
363 | Daily maximum number of international transfers by IBAN reached |
364 | Monthly maximum amount of domestic transfers by IBAN reached |
365 | Monthly maximum amount of international transfers by IBAN reached |
366 | Monthly maximum number of domestic transfers by IBAN reached |
367 | Monthly maximum number of international transfers by IBAN reached |
370 | Customer isn't allowed to ignore thresholds |
501 | Human verification in progress |
602 | Contact the card issuer |
603 | Acceptor invalid |
604 | Keep the card |
605 | Do not honor |
607 | Keep the card, special conditions |
610 | Partially approved (not authorized) |
612 | Transaction invalid |
613 | Amount invalid |
614 | ID cardholder invalid |
615 | Card issuer unknown |
617 | Client cancellation |
619 | Try transaction again later |
620 | Wrong answer (error in server domain) |
621 | No action taken |
624 | Unsupported file update |
625 | Can't find the record within the file |
626 | Duplicate recording, old recording replaced |
627 | Edit error on file update field |
628 | File acces not allowed |
629 | File update impossible |
630 | Format error |
631 | ID acquirer unknown |
632 | Partially completed |
633 | Expiry date pass due |
634 | Fraud suspicion |
638 | Number attempts PIN code exceeded |
641 | Lost card |
643 | Stolen card |
651 | Insufficient funds or exceeded credit limit |
654 | Validity date of card expired |
655 | Wrong PIN |
656 | Card not in the file |
657 | Transaction not allowed for this cardholder |
658 | Prohibited transaction at terminal |
659 | Fraud suspicion |
660 | Card Acceptor must contact the Acquirer |
661 | Withdrawal amount out of range |
663 | Security rules not respected |
668 | Response not received or received too late |
675 | PIN Number attempts exceeded |
676 | Cardholder complaint, please keep all related recording |
690 | Service is temporarily unavailable |
691 | Card issuer inaccessible |
694 | Duplicate request |
696 | System malfunction |
697 | Timing of global monitoring |
698 | Server not available, please try again |
699 | Incident domain initiator |
700 | 3DS service provider error |
6A0 | Contact mode fallback |
6A1 | Non DSP2 compliant transaction rejected (e-commerce only) |
6A2 | PIN requested in single-tap mode |
6A3 | New exchange with authentication required |
6A4 | Improper use of TRA exemptions |
6R1 | Revocation of all recurring payments for this card at merchant |
6R3 | Revocation of all recurring payments for this card |
2001 | Invalid sign date |
2002 | Invalid RUM |
2003 | Signature in progress |
2004 | Signature canceled |
2005 | Signature failed |
2006 | Unknown recycling |
2007 | Invalid recycling status |
2008 | Recycling refused |
2009 | Invalid IBAN BIC |
2010 | Technical reject |
2011 | Bad IBAN threshold |
2012 | Invalid ordering IBAN |
2013 | Unknown SDD |
2014 | Invalid ICS |
2015 | Invalid collection date |
2016 | Unusable bank details |
2017 | Closed account |
2018 | Opposition on account / Blocked account |
2019 | The account holder is a consumer |
2020 | Not allowed operation |
2021 | Invalid operation code |
2022 | Insufficient funds |
2023 | Duplicate |
2024 | Original operation already returned |
2025 | Invalid address |
2026 | Unrecognized transmitter |
2027 | By customer's order |
2028 | Duplicate |
2029 | Invalid Format (Ex MD03) |
2030 | Invalid SDD Type |
2031 | Positive response to Recall |
2032 | Fraudulent Origin Transfer |
2033 | Regulatory reason |
2034 | No authorization / No mandate |
2035 | Incorrect mandate data |
2036 | Contest debtor / Challenge of an authorized transaction |
2037 | Deceased holder |
2038 | By order of the client / Refusal of the debtor |
2039 | Reason not communicated |
2040 | No authorization |
2041 | Received wrongly because original operation not received |
2042 | Incorrect bank code / Bank ID |
2043 | Regulatory reason |
2044 | Regulatory reason |
2045 | Regulatory reason |
2046 | Regulatory reason |
2047 | Specific service |
2048 | Non-compliant SCT due to technical problem |
2049 | Time limit exceeded |
2050 | Unknown BIC |
2051 | Invalid data format |
2500 | Waiting validation |
2501 | To be validated by the head office |
2502 | Have refused by the head office |
2601 | Card Management Service unavailable |
2602 | Incorrect card settings |
2603 | Card not found |
2604 | Invalid carrier card match |
Standing orders
How do I place a standing order?
- Make the first payment via PSP (payment page) or a web service.
- Call the transaction search web service which will give you the alias created for the payment card.
- For the following payments, called the payment web service using the alias as the parameter and not the bank details (card number, expiry date, CVV).
Data dictionary
Please find below a list of spcific fields used in API mode :
Field | Description | Example/values |
---|---|---|
cardType | Card type | CB/VISA/MC/AMEX |
origin | Origin web site of the payment | www.mywebsite.com |
source | Payment source | API/PSP/VPI(eTerminal) |
subAccount | Payment target sub account (marketplace) | SA000001 |
type | Transaction type | D/C/PA/P3F/ALS |
Customer form
With 3D Secure V2, it is strongly advised to fill in as many fields as possible. The form is considered to be one of the most important. The scoring of the transaction will therefore be much better, and will avoid strong authentication, while guaranteeing payment by the cardholder's bank.
- It also allows :
- To find the information of the customer on the portal AfonePayment (detail of an operation, exports Excel)
- The automatic sending of the payment ticket to the mail address of the holder
{ "firstName": "Thomas", "lastName": "Janvier", "email": "tjanvier@comnpay.com", "phone": "+33606060606", "personalPhone": "+33241565858", "professionalPhone": "+33707070707", "road": "tjanvier@comnpay.com", "road2": "Building B", "road3": "Flat B315", "zipCode": "49100", "city": "Angers", "country": "France", "shipRoad": "11 boulevard Maréchal Foch", "shipRoad2": "", "shipRoad3": "", "shipZipCode": "49100", "shipCity": "ANGERS", "shipCountry": "FRANCE", "meetingDate": "20160630180000", "customerRef": "YOURID-ABCD1234" }
Field | Description | Max length |
---|---|---|
firstName | First name | 100 |
lastName | Name | 100 |
Email address | 100 | |
phone | Phone | 20 |
personalPhone | Personal phone | 20 |
professionalPhone | professional phone | 20 |
road | Address | 50 |
road2 | Complementary address | 50 |
road3 | Complementary address | 50 |
zipCode | Zip code | 10 |
city | City | 50 |
country | Country | 50 |
shipRoad | Delivery address | 50 |
shipRoad2 | Complementary delivery address | 50 |
shipRoad3 | Complementary delivery address | 50 |
shipZipCode | Delivery zip code | 10 |
shipCity | Delivery city | 50 |
shipCountry | Delivery country | 50 |
meetingDate | Meeting date Used only for hotels with card verification at D-X before arrival | 14 (format yyyyMMddHHmmss) |
customerRef | Unique customer reference | 40 |
- This form must be sent in JSON
- We advise you to fill in the telephone numbers in international format. This will be particularly useful for SEPA direct debit payments. The telephone number will then already be pre-filled in
Browser
With 3D Secure V2, it is mandatory to fill all fields in browser parameter. Mobile applications are exempt from this setting, but the application parameter is mandatory.
{ "browserAcceptHeader":"Accept: ... Accept-Charset: ... ...", "browserIP": "192.168.14.10", "browserJavaEnabled": true, "browserLanguage": "fr", "browserColorDepth": 24, "browserScreenHeight": 1080, "browserScreenWidth": 1920, "browserTZ": -120, "browserUserAgent": "Mozilla/5.0 (Win...", "challengeWindowSize": "05", "browserJavascriptEnabled": true }
Field | Description | Code |
---|---|---|
browserAcceptHeader | Exact content of the HTTP acceptance | |
browserIP | Browser IP | |
browserJavaEnabled | Represents the ability of the cardholder's browser to run Java | navigator.javaEnabled(); |
browserLanguage | Browser language | navigator.navigator.language(); |
browserColorDepth | Value representing the bit depth of the colour palette for the display | screen.colorDepth; |
browserScreenHeight | screen.height; | |
browserScreenWidth | screen.width; | |
browserTZ | Time difference between UTC time and the local time of the cardholder's browser | getTimezoneOffset(); |
browserUserAgent | HTTP useragent header | |
challengeWindowSize | Dimensions of the challenge window that has been displayed to the cardholder | width x height "01" -> 250 x 400 "02" -> 390 x 400 "03" -> 500 x 600 "04" -> 600 x 400 "05" -> Full screen |
browserJavascriptEnabled | Boolean indicating if I JS is active |
This form must be sent in JSON
Application
With 3D Secure V2, it is mandatory to fill this parameter for mobile applications.
{ "browserIP": "192.168.14.10", "browserLanguage": "fr", }
Field | Description | Code |
---|---|---|
browserIP | Browser IP | |
browserLanguage | Application language |
This form must be sent in JSON
Cardholder Account
With the implementation of 3D Secure V2, it is advised to fill in as many fields as possible.
{ "chAccDate": "20200130", "chAccChange": "20200215", "chAccPwChange": "20201001", "shipAddressUsage": "20200216", "txnActivityDay": 0, "txnActivityYear": 12, "provisionAttemptsDay": 1, "nbPurchaseAccount": 4, "suspiciousAccActivity": "01", "shipNameIndicator": "01" }
- This form must be sent in JSON
- chAccDate : Account opening date
- chAccChange : Date of last account modification
- chAccPwChange : Date of last password change
- shipAddressUsage : Date of first use of the delivery address
- txnActivityDay : Number of transactions (successful and abandoned) on that cardholder's account in the previous 24 hours
- txnActivityYear : Number of transactions (successful and abandoned) for this cardholder's account in the past year
- provisionAttemptsDay : Attempts to add cards to this cardholder's account
- nbPurchaseAccount : Number of purchases (successful transactions) in the last 6 months
- suspiciousAccActivity :
- "01" : No suspicious activity
- "02" : Suspicious activity was observed
- shipNameIndicator :
- "01" : The account name is similar to the delivery name
- "02" : The account name is different from the delivery name
Caddy
With the implementation of 3D Secure V2, it is advised to fill in as many fields as possible.
{ "shipIndicator": "01", "deliveryTimeframe": "01", "deliveryEmailAddress": "tjanvier@comnpay.com", "reorderItemsInd": "01", "preOrderPurchaseInd": "01", "preOrderDate": "20220520", "giftCardAmount": 12, "nbCarteCadeau": 1 }
- This form must be sent in JSON
- shipIndicator : Shipping method chosen for the transaction
- "01" : Send to the cardholder's billing address
- "02" : Send to another address verified by the merchant
- "03" : Send to an address different from the cardholder's billing address
- "04" : "Clic and collect" : Collection from a local shop
- "05" : Digital goods (includes online services, electronic gift cards, ...)
- "06" : Travel and event tickets, undispatched
- "07" : Other (e.g. games, undelivered digital services, electronic media subscriptions, etc.).
- deliveryTimeframe : Delivery time
- "01" : Electronic delivery
- "02" : Same day shipping
- "03" : Overnight shipping
- "04" : Shipping in two or more days
- reorderItemsInd :
- "01" : First order
- "02" : Recommend from the same basket
- preOrderPurchaseInd :
- "01" : Merchandise available
- "02" : Coming soon
- giftCardAmount : For a purchase of prepaid card(s) or gift card(s), the total amount of the purchase of the prepaid card(s) or gift card(s) without the cents (e.g. €12.45 is 12)
- giftCardCount : For the purchase of prepaid cards or gift cards, the total number of prepaid cards or gift cards/codes purchased. The field is limited to 2 characters
Quick example: cURL query in PHP
- cURL is a computer software project providing a library and command-line tool for transferring data using various
- protocols.
The following example initializes the payment for an amount of 10.00 € on the 1111222233334444 card
Do not forget to replace the 'serialNumber' and 'key' fields with your payment identifiers
<?php $webService = 'https://secure.comnpay.com:60000/rest/payment/tokenDebit'; $postFields = array( 'serialNumber' => 'VAD-111-111', 'key' => '6RLl4FlO2o4ZvAdflK2p', 'transactionRef' => 'test', 'tokenRef' => 'DHS73993HEHUD8HJZ' 'amount' => 1000, 'force3ds' => 0, 'ip' => $_SERVER['REMOTE_ADDR'], 'customer' => json_encode(array( "firstName"=>"Thomas", "lastName"=>"Janvier", "email"=>"tjanvier@comnpay.com", "phone"=>"+33606060606", "personalPhone"=>"+33241565858", "professionalPhone"=>"+33707070707", "road"=>"tjanvier@comnpay.com", "road2"=>"Building B", "road3"=>"Flat B315", "zipCode"=>"49100", "city"=>"Angers", "country"=>"France", "shipRoad"=>"11 boulevard Maréchal Foch", "shipRoad2"=>"", "shipRoad3"=>"", "shipZipCode"=>"49100", "shipCity"=> "ANGERS", "shipCountry"=> "FRANCE", "meetingDate"=>"20160630180000", "customerRef"=>"YOURID-ABCD1234" )), 'browser' => json_encode(array( "browserAcceptHeader":"Accept: ... Accept-Charset: ... ...", "browserIP"=>"192.168.14.10", "browserJavaEnabled"=>true, "browserLanguage"=>"fr", "browserColorDepth"=>24, "browserScreenHeight"=>1080, "browserScreenWidth"=>1920, "browserTZ"=>-120, "browserUserAgent"=>"Mozilla/5.0 (Wi...", "challengeWindowSize"=>"05", "browserJavascriptEnabled"=>true, )), 'cardholderAccount' => json_encode(array( "chAccDate"=>"20200130", "chAccChange"=>"20200215", "chAccPwChange"=>"20201001", "shipAddressUsage"=>"20200216", "txnActivityDay"=>0, "txnActivityYear"=>12, "provisionAttemptsDay"=>1, "nbPurchaseAccount"=>4, "suspiciousAccActivity"=>"01", "shipNameIndicator"=>"01", )), 'caddy' => json_encode(array( "shipIndicator"=>"01", "deliveryTimeframe"=>"01", "deliveryEmailAddress"=>"tjanvier@comnpay.com", "reorderItemsInd"=>"01", "preOrderPurchaseInd"=>"01", "preOrderDate"=>"20220520", "giftCardAmount"=>12, "nbCarteCadeau"=>1 )) ); $datasString = http_build_query($postFields); $ch = curl_init(); curl_setopt($ch,CURLOPT_URL, $webService); curl_setopt($ch,CURLOPT_POSTFIELDS, $datasString); $result = curl_exec($ch); curl_close($ch); echo $result; ?>
Test cards
Here are the cards that can be used to simulate the various payment in our homologation platform:
Without 3D Secure: Any payment strictly less than 30€.
For any payment superior or equal to 30€:
- 3D Secure V1: 2221001892683407
- 3D Secure V2 with authentication: 5306889942833340 > The code to be entered in the authentication page is 1234
- 3D Secure V2 without authentication ("frictionless"): 5512459816707530